How to create Dynamic Breadcrumbs using “react-router-dynamic-breadcrumbs”
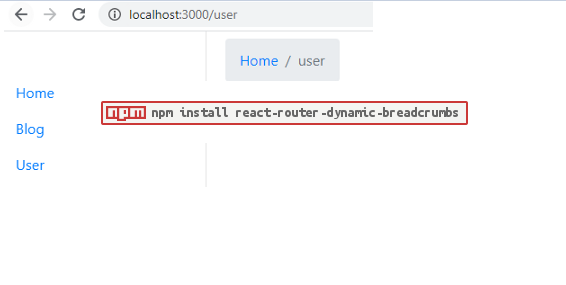
Hello all !!! . Today, I show you how to make the breadcrumbs in your site function super easily. The magic is using Dynamic Breadcrumbs.
So, we’ll complete the following steps in this tutorial,
- Create a sidebar, header, breadcrumb for the app
- Set some routes for demonstration (Like /home, /user, etc..)
- Use react-router-dynamic-breadcrumbs module and render the breadcrumbs
In a previous tutorial, I showed you how to start a React+Typescript app from scratch. So, here I’m not going to cover that part. Please take a look at it and create your app.
Let’s get dirty your hands.
Under the component folder, create these files.
- Sidebar.tsx
- Header.tsx.
- Dashboard.css (you can place this in another folder)
- AppBreadCrumbs.tsx
Note that you have to install the necessary packages/modules that are imported in these classes by tying as follows in the terminal.
npm install package_name
When importing the modules, you might face an error like “could not find a declaration file for module …”. You can fix that error by adding “noImplicitAny”: false under “compilerOptions” in file tsconfig.json.
This is my sidebar which is so simple, but it will do the job. I added a .css file just to make it look better. In my sidebar, there are 3 links for Blog, User and New User X pages. With href = “/”, I have set the relevant routes for each page.
//Sidebar.tsximport React from "react";
import {Nav} from "react-bootstrap";
import './Dashboard.css'export class Sidebar extends React.Component {
render(){
return (
<>
<Nav className="col-md-2 d-md-block sidebar" activeKey="/home" >
<Nav.Item>
<Nav.Link href="/blog">Blog</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link href="/user">User</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link href="/user/new/x">New User X</Nav.Link>
</Nav.Item>
</Nav>
</>
);
}
};export default Sidebar;
Here, is the stylish sheet for my sidebar.
//Dashboard.css.sidebar {
position: fixed;
top: 0;
bottom: 0;
left: 0;
min-height: 100vh !important;
z-index: 100;
padding: 48px 0 0;
box-shadow: inset -1px 0 0 rgba(0, 0, 0, .1);
}
Okay, the other file that needs to be created is Header.tsx. You might have noticed before, that most of the breadcrumbs are located inside the header. So, we’ll create a breadcrumb component next and include it in our header component.
//Header.tsximport React from "react";
import { AppBreadcrumbs } from "./AppBreadCrumbs";
import { Row, Col } from 'reactstrap';export class Header extends React.Component {
render(){
return <div className="header navbar">
<div className="header-container">
<Row >
<Col >
<AppBreadcrumbs/>
</Col>
</Row>
</div>
</div>
}
}
Now, let’s create our most important component which is, AppBreadCrumbs.tsx.
//AppBreadCrumbs.tsx.import React from "react";
import Breadcrumbs from 'react-router-dynamic-breadcrumbs';
import {BrowserRouter as Router} from 'react-router-dom';const routesList = {
'/': 'Home',
'/blog': 'Blog',
'/user': 'User',
'/user/new/:id': 'User Info',
}export class AppBreadcrumbs extends React.Component {
render() {
return (
<Router>
<Breadcrumbs mappedRoutes={routesList}
WrapperComponent={(props: any) =>
<ol className="breadcrumb">{props.children}</ol>}
ActiveLinkComponent={(props: any) =>
<li className="breadcrumb-item active" >{props.children}</li>}
LinkComponent={(props: any) =>
<li className="breadcrumb-item">{props.children}</li>
} />
</Router>
);
}
}
We have created a routesList which includes our routes list (the links our app navigates to). Inside the class, I have rendered the BreadCrumbs. Our routesList is set as the mappedRoutes. MappedRoutes should be a plain javascript object with routes paths and names/resolver callbacks. The WrapperComponent is the function that creates the wrapper html structure. The ActiveLinkComponent creates the active link html structure while the LinkComponent creates the link html structure of the breadcrumb path.
Okay, before running the app, you have to make some changes to App.tsx file which comes default when you create the app.
//App.tsximport React from 'react';
import './App.css';
import 'bootstrap/dist/css/bootstrap.min.css';
import Sidebar from './components/Sidebar';
import { Row, Col } from 'react-bootstrap';
import { Header } from './components/Header';function App() {
return (
<>
<div className='wrapper'>
<Row>
<Col xs={2} >
<Sidebar />
</Col>
<Col xs={10}>
<Header/>
</Col>
</Row>
</div>
</>
);
}export default App;
Let’s run the app by typing npm start in the terminal and hit enter.

This is what you will see as the start page. When you click the menus in the sidebar, you will see the URL is changing according to as in the routesList and also you will see the dynamic breadcrumb that automatically creates.
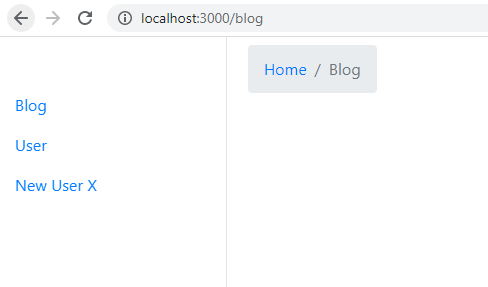


That’s all folks !!!. I hope you learn something. Happy coding 😁
References:
https://www.npmjs.com/package/react-router-dynamic-breadcrumbs